Rust on microcontrollers
Instructor: Michael Kefeder
First we’ll look at how to write a minimalistic I2C driver for a touchpanel of a small e-paper display, to have an easy introduction into the basic concepts of the Rust embedded eco-system.
Then we’ll inspect the implementation of a driver for a SPI controlled transceiver module MRF89XA to see which techniques and libraries can be useful. We will use the manufacturer provided specifications sheet and their example C code to improve the existing driver with Rust. Using the driver we’ll see how one can write a minimal application that communicates with other microcontrollers wirelessly. Additionally we’ll discover how to write Rust code that is compatible with the widely used over the air protocol RadioHead embedded packet radio software (GPL licensed). The workshop includes hands-on exercises applying the learnt concepts.
Prerequisites / target audience
- low-level programmers in the embedded space using C, C++ or Rust.
- experienced Rust programmers without embedded background
If you have neither experience with microcontrollers nor with Rust following this workshop will be very hard. You’ll have to then learn the concepts behind embedded programming and a new programming language simultaneously, not impossible, but potentially too much.
Topics
- bitfields, like in C, but compile time verified
no_std
writing code for systems without OS and standard library- Rust embedded terminology like HAL, PAC, BSP
- examples how to communicate over i2c and SPI
- translate C
#define
macros into something sane and compile time verifyable - ways how to implement a binary communications protocol with Rust, that was originally written in a different language (here C++)
- tooling for writing, flashing and debugging embedded software on microcontrollers
- how to write tests for a
no_std
crate and run them on a computer with standard library available
Technological prerequisites
Hardware
- laptop computer you can write and compile Rust code on (see software requirements below)
- minimum 2x RP2040 based microcontroller boards with pins for a breadboard
- e.g. Raspberry Pi Pico, pre-soldered pins from Seeed Studio
- one will be used as a debugger for the other, alternatively you can bring a Raspberry Pi Debug Probe
- breadboard(s) for both Pico’s or Debug probe
- breadboard jumper cables or Debug probe (the latter comes with needed cables)
- USB cables matching your setup
- USB hub (likely needed to accomodate USB-micro plug devices)
Nice to have hardware
- since we implement wireless communication, it helps to have 3x RP2040: one running the receiver software while we debug with the 2 others on the sender software
- 2x MRF89XAM8A-I/RM radio modules, solder pins on them for your breadboard(!)
- a few of those modules will be provided by the workshop host, and are enough to test your code, but the experience will be more interactive when you have your own to play with
- breadboards big enough to hold the Pico’s and the radio modules
Software:
Please bring your computer pre-installed with the following tools:
- rustc 1.76 or newer installed via rustup
- rustfmt
rustup component add rustfmt
- clippy
rustup component add clippy
- embedded programming tools for cross-compiling, linking and flashing onto the RP2040
# install compilation targets
rustup target add thumbv6m-none-eabi
rustup target add --toolchain nightly thumbv6m-none-eabi
# necessary linker
cargo install flip-link
# Useful to creating UF2 images for the RP2040 USB Bootloader
cargo install elf2uf2-rs --locked
# Useful for flashing over the SWD pins using a supported JTAG probe
# this contains cargo-embed too. Also available via homebrew
cargo install cargo-binstall
cargo binstall probe-rs-tools
- for more information about installing the probe-rs-tools see their documentation https://probe.rs/docs/getting-started/installation/
- finally you can clone this repository and see if the project in
rp2040-blink
compiles and runs on your RP2040 https://github.com/bedroombuilds/pico-usb
It is strongly advised to use Linux, macOS or WSL on Windows. Pure Windows setups work for sure too, but from experience cross-compiling on there is harder to achieve.
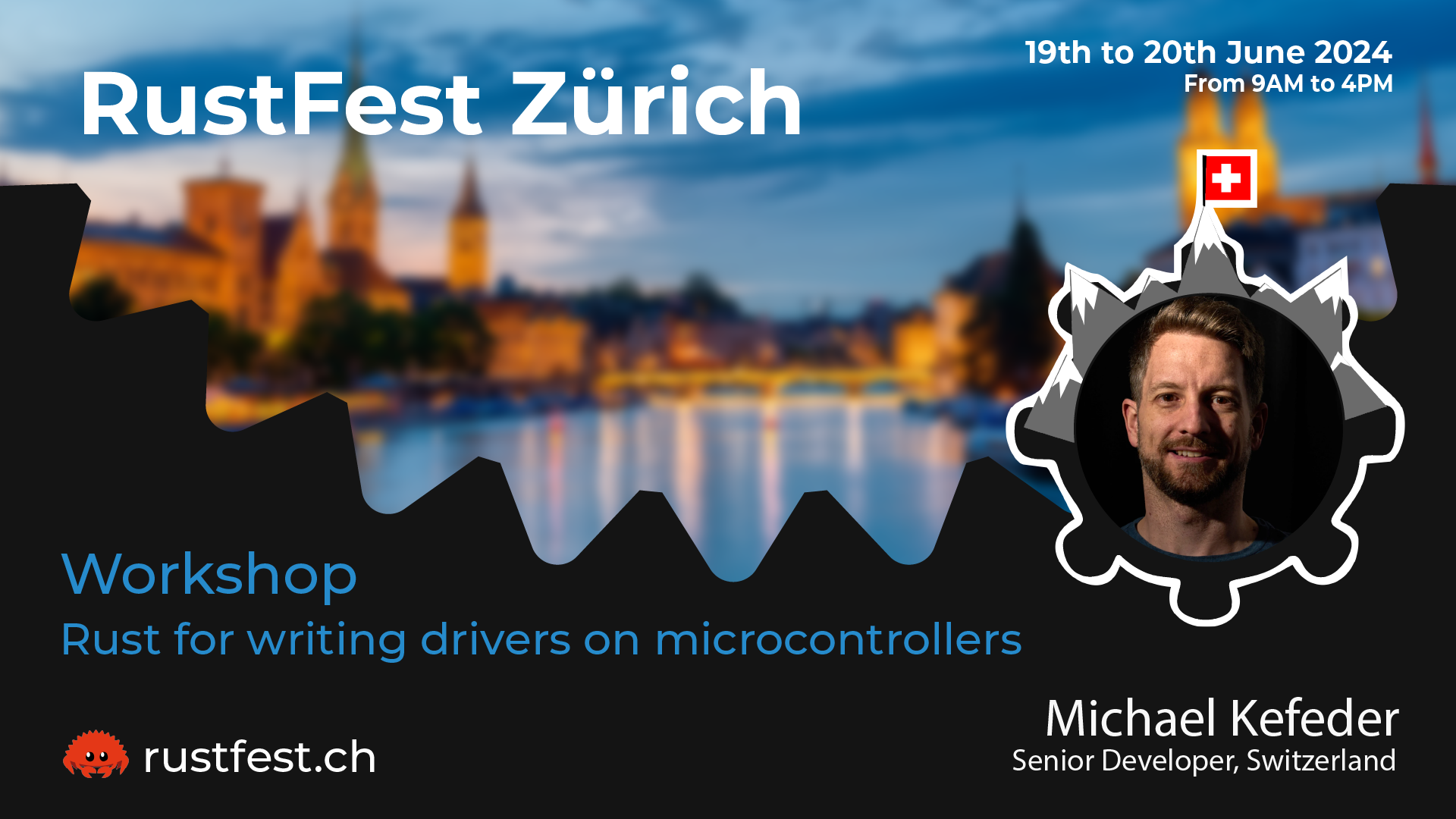
For location and schedule visit our Workshops page